The definition of linting on Wikipedia is pretty clear :”Lint, or a linter, is a tool that analyzes source code to flag programming errors, bugs, stylistic errors, and suspicious constructs”.
By using a linter in your React Native project you’ll be able to catch code errors early on and correct them before it’s too late. For a React Native project, we will rely on ESLint, and this is how we are going to do it:
1. Install the linter plugin for your IDE
I am using VSCode and a great Linter plugin is ESLint. There are usually several plugin alternatives for the most common IDEs, go and grab your favorite.
2. Install ESLint
The eslint package is the tool that will check for errors in our code. Open the command line, switch to your React Native project folder and run:
npm install eslint --save-dev
3. Install the Babel parser
We need the Babel parser to allow ESLint to check for code written using the ES7 syntax, Flow or TypeScript types.
npm install babel-eslint --save-dev
4. Get a list of rules for ESLint
For ESLint to properly work we need to configure ESLint with a list of rules. You can write the instructions yourself but there are already existing solutions, so we will just pick one of them.
Here are a few:
Airbnb JavaScript Style Guide() {
ESLint-plugin-React
ESLint plugin for React Native
We will continue with the esling-plugin-react-native. To install it, run:
npm install eslint-plugin-react-native --save-dev
5. Add the .eslintrc file to your project
To configure ESLint we need to create a .eslintrc file at the root of the project. Open your text editor and add the following to your .eslintrc file:
{ "plugins": [ "react-native" ], "extends": [ "eslint:recommended", "plugin:react-native/all" ], "parser": "babel-eslint", "env": { "react-native/react-native": true }, "parserOptions": { "ecmaFeatures": { "jsx": true } }, "rules":{ "react-native/no-unused-styles": 2, "react-native/split-platform-components": 2, "react-native/no-inline-styles": 2, "react-native/no-color-literals": 2, "react-native/no-raw-text": 2 } }
As soon as you save your .eslintrc file, you should see a lot of errors showing up in your code. No worries, these are probably some styling or import related issues, go ahead and fix them. You can now enjoy you clean code😉
We can work together
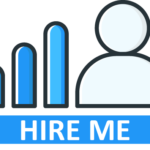
Share this post on social media: