There are already several tutorials of that kind available on the Internet. However, I couldn’t follow any of them from the begining to the end without issues, so here is my own tutorial about how to write and compile your very first smart contract for the Ethereum blockchain. This first article is all about the Dapp code and compiling, a second article about how to deploy our app on the network will follow.
A smart contract corresponds to code instructions that are supposed to run on the network. In the case of Ethereum, a runtime environment called EVM (Ethereum Virtual Machine) takes care of running smart contracts. The instructions of the smart contract are written using the Solidity programming language. There are 2 essential steps to successfully run a smart contract on the network: compiling and deploying. Compiling the smart contract will generate a bitcode that can be later deployed on the network. So, let’s get ready to write our first smart contract!
Required tools
The only tools that you need to follow this tutorial are Node.js and npm installed in your machine.
1. Initialize a new node project
Open your terminal and create a new package.json using the npm command line tool:
npm init helloWorldContract
Confirm all the steps by pressing Enter or adapt the answers if needed. This will create a new package.json file containing all the information provided above.
2. Install solc
The npm solc package is a JavaScript library for the solidity compiler. We will use the solc package to compile our smart contract so that it is ready to be deployed on the network.
npm i solc
3. Write the smart contract
The next step is to write our smart contract. There are several essential points to write a smart contract properly:
- a smart contract always starts with the “pragma solidity ^0.5.2;” definition, where 0.5.2 is the version of the Solidity compiler
- a smart contract is defined by the “contract” keyword followed by its name
- a list of variables and functions defines what the smart contract is meant to do
Copy paste the code below to your text editor and save the file as Hello.sol
pragma solidity ^0.5.2; contract HelloWorld { uint256 counter = 5; function increment() public { counter++; } function decrement() public { counter--; } }
In this example we declared a counter variable of type uint256 and initialized it to 5. We then defined 2 functions in our smart contract; the first one to increment our counter and the second one to decrement it.
4. Write the compiler code
We now need to write the JavaScript code that will compile our smart contract. Create a new file, save it as compileSol.js and add the following code to it
const path = require('path'); const fs = require('fs'); const solc = require('solc'); const helloPath = path.resolve(__dirname, 'Hello.sol'); const hellosol = fs.readFileSync(helloPath, 'UTF-8'); var input = { language: 'Solidity', sources: { 'hello.sol': {content : hellosol} }, settings: { outputSelection: { '*': { '*': [ '*' ] } } } }; var output = JSON.parse(solc.compile(JSON.stringify(input))) for (var contractName in output.contracts['hello.sol']) { console.log(contractName + ': ' + output.contracts['hello.sol'][contractName].evm.bytecode.object) }
5. Compile
Run the compiler code on your terminal as below
node compileSol.js
The terminal will show you the generated bitcode corresponding to our HelloWorld smart contractas below:
HelloWorld: 6080604052600560005534801561001557600080fd5b5060b7806100246000396000f3fe6080604052348015600f57600080fd5b5060043610604f576000357c0100000000000000000000000000000000000000000000000000000000900480634f2be91f1460545780636deebae314605c575b600080fd5b605a6064565b005b60626077565b005b6000808154809291906001019190505550565b60008081548092919060019003919050555056fea165627a7a7230582010eb14dc210d8b3d190848ce79ded65e30d7502c032655c7889d2fa7d35d3f1d0029
This is what we will need to publish our smart contract to the network. I will explain more in details about how to publish the contract on the network in the next blogpost of the series.
We can work together
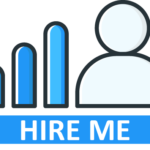
Share this post on social media: